とりあえず、出来上がったコードを張っておきます。(動作確認レベルで、リファクタリングすらしてない)
必要なライブラリなどは後で解説します
2022/5/4更新
上から順にコード詰め込んだだけなので、汚いですが。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 |
from bs4 import BeautifulSoup import urllib.request as req import requests import lxml.html import re import pandas as pd import gspread from gspread_dataframe import set_with_dataframe from oauth2client.service_account import ServiceAccountCredentials #GoogleからもらったJSONファイルで、スコープのAPIが使えるように認証情報を取得 SCOPES = ['https://spreadsheets.google.com/feeds','https://www.googleapis.com/auth/drive'] SERVICE_ACCOUNT_FILE ='秘密鍵情報はgoogleAPIから取得しよう!.json' credentials = ServiceAccountCredentials.from_json_keyfile_name(SERVICE_ACCOUNT_FILE, SCOPES) #認証情報を使って、スプレッドシート の操作券を取得 gs = gspread.authorize(credentials) #共有したスプレッドシート のキー(スプレッドシート のURLの真ん中ら辺)を使ってシートの情報を取得 SPREADSHEET_KEY = '書き込みたいスプレッドシート のURL' worksheet = gs.open_by_key(SPREADSHEET_KEY).worksheet('書き込みたいシートの名前を入れる') #値がとれてるかとりあえずプリントしてみる print(worksheet.acell('R9').value) workbook = gs.open_by_key(SPREADSHEET_KEY) worksheet = workbook.worksheet('12') print(workbook.title) print(workbook.id) print(worksheet) df = pd.DataFrame(worksheet.get_all_values()) df.head() #行と列の数を取り出す sh=df.shape #shのprint表示 print(sh) print(sh[1]) #shapeの列の数だけrangeで振り直してcolumnsに代入 df.columns=range(sh[1]) df.head() df.dtypes #ここで数字以外は空白にする 空白は次のreplace,dropnaで消す df[2]= df[2].str.replace('仕入額','') df[2]= df[2].str.replace(',','') df[2]= df[2].str.replace('¥','') df[2]= df[2].str.replace('/','') #df[2]= df[2].str.replace(r'\D+','') #df[2]= df[2].str.replace(r'[^0-9]+','') print(df[8:9][2]) import numpy as np #空白セルを一旦npnに置き換え numpyライブラリで、NaNと一旦置き換え df[2].replace('',np.nan,inplace=True) print(df[8:9][2]) #NaNのセル行ごと削除 df.dropna(subset=[2],inplace=True) print(df[8:9][2]) pd.set_option("display.max_rows", 50) print(df[8:9]) print(df[8:9][2]) print(df[2]) df[2] =df[2].astype(int) df.dtypes pd.set_option("display.max_rows", 500) print(df[2]) df_sum = df[2].sum() df_sum workbook.add_worksheet(title='うんち2',rows=100,cols=100) print(df_sum) asin = input() print(asin) deltaUrl ='https://delta-tracer.com/item/detail/jp/'+asin print(deltaUrl) response = req.urlopen(deltaUrl) parse_html = BeautifulSoup(response,'html.parser') print(parse_html.title.string) print(parse_html.find_all('a')) print(parse_html.select('.item_img-large')) url = 'https://delta-tracer.com/item/detail/jp/'+asin print(url) response = requests.get(url) print(response.text) html = lxml.html.fromstring(response.content) print(html) htmlpn = html.xpath("/html/body/section/div[2]/div[2]/div[2]/div[1]/div/table/tbody/tr/td[2]/div/a[1]/strong") print(htmlpn[0].text) htmlasin = html.xpath("//*[@class='selectable']") print("ASIN: "+htmlasin[0].value) htmlasin = html.xpath("//*[@class='selectable']") print("JAN: "+htmlasin[1].value) htmlogata = html.xpath("/html/body/section/div[2]/div[2]/div[2]/div[1]/div/table/tbody/tr/td[2]/div/span[1]/span[1]") print("大型商品: "+htmlogata[0].text) html1 = html.xpath("/html/body/section/div[2]/div[2]/div[2]/div[1]/div/div[2]/table/tbody[2]/tr[1]/td[4]/span") print(html1) for item1 in html1: print(item1.text()) print(item1.text_content()) htmlrank = html.xpath("/html/body/section/div[2]/div[2]/div[2]/div[1]/div/table/tbody/tr/td[2]/div/span[2]/span/strong") print('ランキング: '+htmlrank[0].text+"位") htmlsho = html.xpath("//*[@class='text-right']") print('出品数: '+htmlsho[5].text_content()+"\n") Nseller = htmlsho[5].text_content() #print(htmlsho) #for item in htmlsho: # print(item.text_content()) #text = td.text() #text = td.text_content() Nseller = re.sub("\\D", "", Nseller) print('出品数: '+Nseller+"件\n") #reモジュールによって文字列から数字のみを抜き出した #一つ前の行だけだと、文字列と数字と空白が合体してしまってる parse_lists=parse_html.find_all('strong') parse_lists[1:100] parse_lists[7].string strong_list=[] for i in parse_lists: strong_list.append(i.string) strong_list #データフレーム 作り df_strong_list = pd.DataFrame({'タグのみ抜き出した時':parse_lists,'文字列のみに変換した時':strong_list}) df_strong_list #行に1つでも欠損値(NaN)があればその行消す。anyが1つでも、allが行全て df_notnull = df_strong_list.dropna(how='any') df_notnull #特定の文字列を含むか判定str.contains \dで数字全てってことの正規表現。要は、数字入ってるとこだけ抽出 df_notnull['文字列のみに変換した時'].str.contains('\d') #上の判定結果を[]の中に入れると、Trueの行だけ表示される df_notnull[df_notnull['文字列のみに変換した時'].str.contains('\d')] #書き出しように、変数に代入 df_contain_python =df_notnull[df_notnull['文字列のみに変換した時'].str.contains('\d')] set_with_dataframe(workbook.worksheet('うんち2'), df_contain_python, include_index=True) |
Google APIでMyProjectを作って、スプレッドシート操作用のキーを取得する
ライブラリのインストール
pipコマンドで以下をインストール
BeautifulSoup
urllib.request
requests
lxml
re
pandas
gspread
gspread_dataframe
oauth2client.service_account
入れたかわかんなくなったら、
pip show ***** **にはライブラリ名を入れる
今日はここまでにして、続きは後日書きます。以下の画像は動いてる姿。
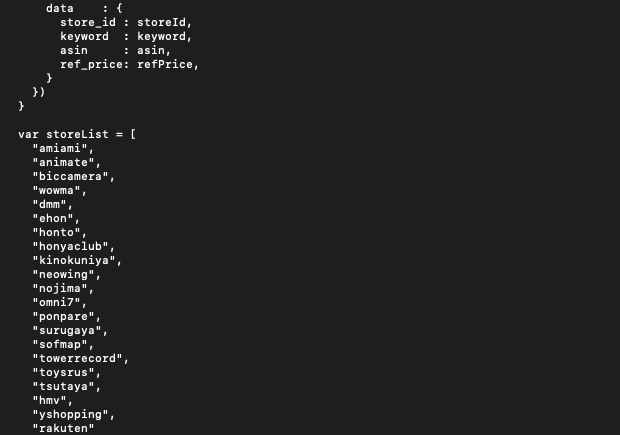